6. API - pi-top Maker Architecture (PMA) Components
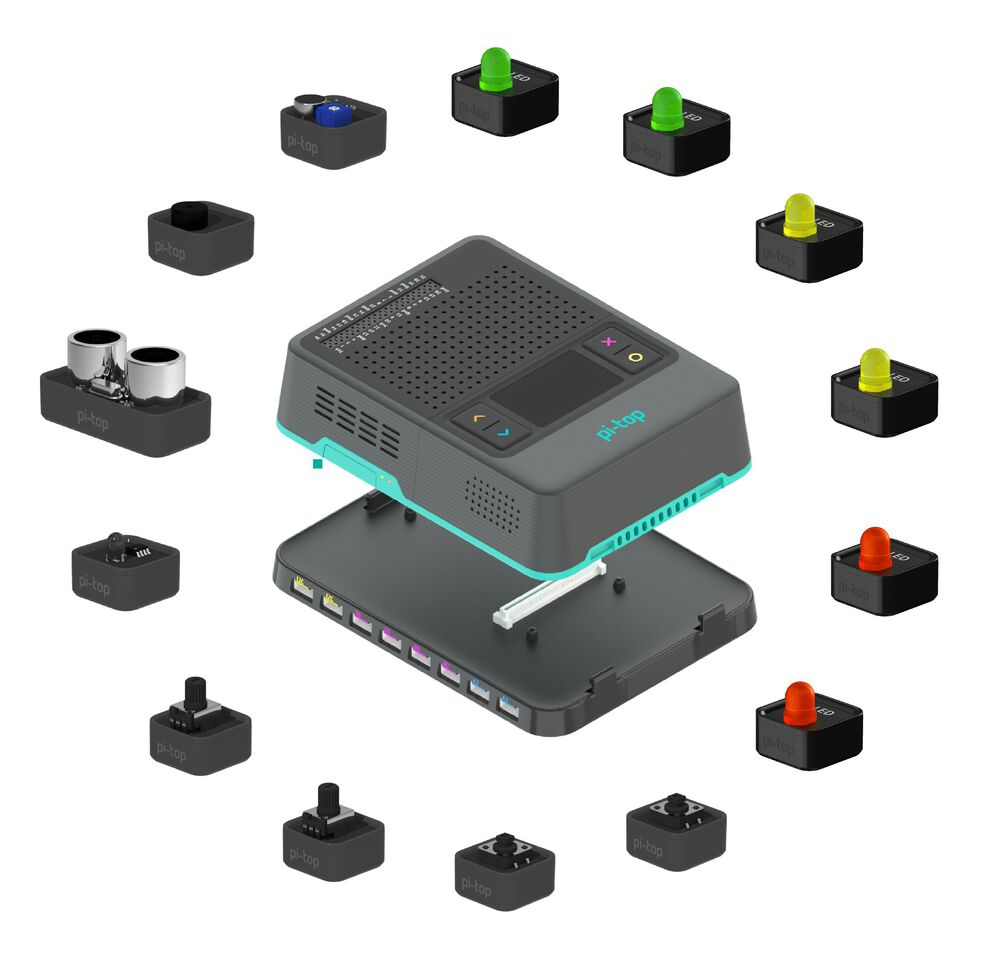
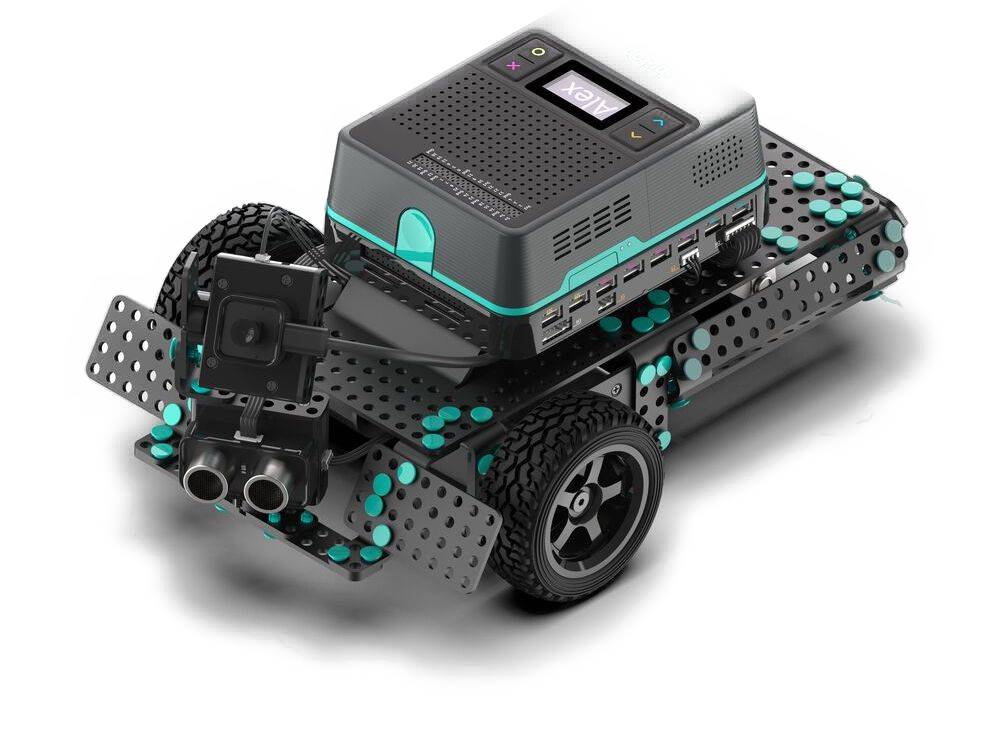
The Foundation & Expansion Plates and all the parts included in the Foundation & Robotics Kit are known as the pi-top Maker Architecture (PMA).
Each PMA component has a Python class provided for it.
Check out Key Concepts: pi-top Maker Architecture for useful information to get started with using PMA.
6.2. Buzzer
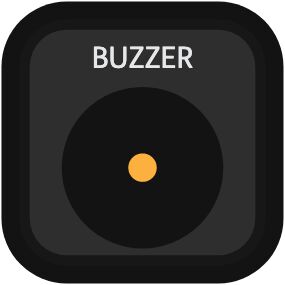
Note
This is a Digital Component which connects to a Digital Port [D0-D7].
from time import sleep
from pitop import Buzzer
buzzer = Buzzer("D0")
buzzer.on() # Set buzzer sound on
print(buzzer.value) # Return 1 while the buzzer is on
sleep(2)
buzzer.off() # Set buzzer sound off
print(buzzer.value) # Return 0 while the buzzer is off
sleep(2)
buzzer.toggle() # Swap between on and off states
print(buzzer.value) # Return the current state of the buzzer
sleep(2)
buzzer.off()
- class pitop.pma.Buzzer(*args, **kwargs)[source]
Encapsulates the behaviour of a simple buzzer that can be turned on and off.
- Parameters:
port_name (str) – The ID for the port to which this component is connected
name (str) – Component name, defaults to buzzer. Used to access this component when added to a
pitop.Pitop
object.
- property own_state
Representation of an object state that will be used to determine the current state of an object.
- close()[source]
Shut down the device and release all associated resources. This method can be called on an already closed device without raising an exception.
This method is primarily intended for interactive use at the command line. It disables the device and releases its pin(s) for use by another device.
You can attempt to do this simply by deleting an object, but unless you’ve cleaned up all references to the object this may not work (even if you’ve cleaned up all references, there’s still no guarantee the garbage collector will actually delete the object at that point). By contrast, the close method provides a means of ensuring that the object is shut down.
For example, if you have a buzzer connected to port D0, but then wish to attach an LED instead:
>>> from pitop import Buzzer, LED >>> bz = Buzzer("D0") >>> bz.on() >>> bz.off() >>> bz.close() >>> led = LED("D0") >>> led.blink()
Device
descendents can also be used as context managers using thewith
statement. For example:>>> from pitop import Buzzer, LED >>> with Buzzer("D0") as bz: ... bz.on() ... >>> with LED("D0") as led: ... led.on() ...
- property active_high
When
True
, thevalue
property isTrue
when the device’spin
is high. WhenFalse
thevalue
property isTrue
when the device’s pin is low (i.e. the value is inverted).This property can be set after construction; be warned that changing it will invert
value
(i.e. changing this property doesn’t change the device’s pin state - it just changes how that state is interpreted).
- beep(on_time=1, off_time=1, n=None, background=True)
Make the device turn on and off repeatedly.
- Parameters:
on_time (float) – Number of seconds on. Defaults to 1 second.
off_time (float) – Number of seconds off. Defaults to 1 second.
n (int or None) – Number of times to blink;
None
(the default) means forever.background (bool) – If
True
(the default), start a background thread to continue blinking and return immediately. IfFalse
, only return when the blink is finished (warning: the default value of n will result in this method never returning).
- blink(on_time=1, off_time=1, n=None, background=True)
Make the device turn on and off repeatedly.
- Parameters:
on_time (float) – Number of seconds on. Defaults to 1 second.
off_time (float) – Number of seconds off. Defaults to 1 second.
n (int or None) – Number of times to blink;
None
(the default) means forever.background (bool) – If
True
(the default), start a background thread to continue blinking and return immediately. IfFalse
, only return when the blink is finished (warning: the default value of n will result in this method never returning).
- property closed
Returns
True
if the device is closed (see theclose()
method). Once a device is closed you can no longer use any other methods or properties to control or query the device.
- property config
Returns a dictionary with the set of parameters that can be used to recreate an object.
- static ensure_pin_factory()
Ensures that
Device.pin_factory
is set appropriately.This is called implicitly upon construction of any device, but there are some circumstances where you may need to call it manually. Specifically, when you wish to retrieve board information without constructing any devices, e.g.:
Device.ensure_pin_factory() info = Device.pin_factory.board_info
If
Device.pin_factory
is notNone
, this function does nothing. Otherwise it will attempt to locate and initialize a default pin factory. This may raise a number of different exceptions includingImportError
if no valid pin driver can be imported.
- classmethod from_config(config_dict)
Creates an instance of a Recreatable object with parameters in the provided dictionary.
- classmethod from_file(path)
Creates an instance of an object using the JSON file from the provided path.
- static import_class(module_name, class_name)
Imports a class given a module and a class name.
- property is_active
Returns
True
if the device is currently active andFalse
otherwise. This property is usually derived fromvalue
. Unlikevalue
, this is always a boolean.
- off()
Turns the device off.
- on()
Turns the device on.
- property pin
The
Pin
that the device is connected to. This will beNone
if the device has been closed (see theclose()
method). When dealing with GPIO pins, querypin.number
to discover the GPIO pin (in BCM numbering) that the device is connected to.
- print_config()
- print_state()
- save_config(path)
Stores the set of parameters to recreate an object in a JSON file.
- property source_delay
The delay (measured in seconds) in the loop used to read values from
source
. Defaults to 0.01 seconds which is generally sufficient to keep CPU usage to a minimum while providing adequate responsiveness.
- property state
Returns a dictionary with the state of the current object and all of its children.
- toggle()
Reverse the state of the device. If it’s on, turn it off; if it’s off, turn it on.
- property value
Returns 1 if the device is currently active and 0 otherwise. Setting this property changes the state of the device.
6.3. Encoder Motor
Note
This is a Motor Component which connects to a MotorEncoder Port [M0-M3].
from time import sleep
from pitop import BrakingType, EncoderMotor, ForwardDirection
# Setup the motor
motor = EncoderMotor("M0", ForwardDirection.COUNTER_CLOCKWISE)
motor.braking_type = BrakingType.COAST
# Move in both directions
rpm_speed = 100
for _ in range(4):
motor.set_target_rpm(rpm_speed)
sleep(2)
motor.set_target_rpm(-rpm_speed)
sleep(2)
motor.stop()
- class pitop.pma.EncoderMotor(port_name, forward_direction, braking_type=BrakingType.BRAKE, wheel_diameter=0.07, name='encoder_motor')[source]
Represents a pi-top motor encoder component.
Note that pi-top motor encoders use a built-in closed-loop control system, that feeds the readings from an encoder sensor to an PID controller. This controller will actively modify the motor’s current to move at the desired speed or position, even if a load is applied to the shaft.
This internal controller is used when moving the motor through
set_target_rpm
orset_target_speed
methods, while using theset_power
method will make the motor work in open-loop, not using the controller.Note
Note that some methods allow to use distance and speed settings in meters and meters per second. These will only make sense when using a wheel attached to the shaft of the motor.
The conversions between angle, rotations and RPM used by the motor to meters and meters/second are performed considering the
wheel_diameter
parameter. This parameter defaults to the diameter of the wheel included with MMK. If a wheel of different dimmensions is attached to the motor, you’ll need to measure it’s diameter, in order for these methods to work properly.- Parameters:
port_name (str) – The ID for the port to which this component is connected.
forward_direction (ForwardDirection) – The type of rotation of the motor shaft that corresponds to forward motion.
braking_type (BrakingType) – The braking type of the motor. Defaults to coast.
wheel_diameter (int or float) – The diameter of the wheel attached to the motor.
name (str) – Component name, defaults to encoder_motor. Used to access this component when added to a
pitop.Pitop
object.
- property own_state
Representation of an object state that will be used to determine the current state of an object.
- property forward_direction
Represents the forward direction setting used by the motor.
Setting this property will determine on which direction the motor will turn whenever a movement in a particular direction is requested.
- Parameters:
forward_direction (ForwardDirection) – The direction that corresponds to forward motion.
- property braking_type
Returns the type of braking used by the motor when it’s stopping after a movement.
Setting this property will change the way the motor stops a movement:
BrakingType.COAST
will make the motor coast to a halt when stopped.BrakingType.BRAKE
will cause the motor to actively brake when stopped.
- Parameters:
braking_type (BrakingType) – The braking type of the motor.
- set_power(power, direction=Direction.FORWARD)[source]
Turn the motor on at the power level provided, in the range -1.0 to.
+1.0, where:
1.0: motor will turn with full power in the
direction
provided as argument.0.0: motor will not move.
-1.0: motor will turn with full power in the direction contrary to
direction
.
Warning
Setting a
power
value out of range will cause the method to raise an exception.
- power()[source]
Get the current power of the motor.
Returns a value from -1.0 to +1.0, assuming the user is controlling the motor using the
set_power
method (motor is in control mode 0). If this is not the case, returns None.
- set_target_rpm(target_rpm, direction=Direction.FORWARD, total_rotations=0.0)[source]
Run the motor at the specified
target_rpm
RPM.If desired, a number of full or partial rotations can also be set through the
total_rotations
parameter. Once reached, the motor will stop. Settingtotal_rotations
to 0 will set the motor to run indefinitely until stopped.If the desired RPM setting cannot be achieved,
torque_limited
will be set toTrue
and the motor will run at the maximum possible RPM it is capable of for the instantaneous torque. This means that if the torque lowers, then the RPM will continue to rise until it meets the desired level.Care needs to be taken here if you want to drive a vehicle forward in a straight line, as the motors are not guaranteed to spin at the same rate if they are torque-limited.
Warning
Setting a
target_rpm
higher than the maximum allowed will cause the method to throw an exception. To determine what the maximum possible target RPM for the motor is, use themax_rpm
method.
- target_rpm()[source]
Get the desired RPM of the motor output shaft, assuming the user is controlling the motor using
set_target_rpm
(motor is in control mode 1).If this is not the case, returns None.
- property current_rpm
Returns the actual RPM currently being achieved at the output shaft, measured by the encoder sensor.
This value might differ from the target RPM set through
set_target_rpm
.
- property rotation_counter
Returns the total or partial number of rotations performed by the motor shaft.
Rotations will increment when moving forward, and decrement when moving backward. This value is a float with many decimal points of accuracy, so can be used to monitor even very small turns of the output shaft.
- property torque_limited
Check if the actual motor speed or RPM does not match the target speed or RPM.
Returns a boolean value,
True
if the motor is torque- limited andFalse
if it is not.
- property max_rpm
Returns the approximate maximum RPM capable given the motor and gear ratio.
- property wheel_diameter
Represents the diameter of the wheel attached to the motor in meters.
This parameter is important if using library functions to measure speed or distance, as these rely on knowing the diameter of the wheel in order to function correctly. Use the predefined pi-top wheel measurement, or define your own wheel size.
Note
The wheel diameter changes slightly when in contact with a surface due to rubber compression.
For this application, the key measurement is the diameter when the wheel rests on a flat surface. Based on this, the default wheel diameter used is 0.070m.
- property wheel_circumference
- set_target_speed(target_speed, direction=Direction.FORWARD, distance=0.0)[source]
Run the wheel at the specified target speed in meters per second.
If desired, a
distance
to travel can also be specified in meters, after which the motor will stop. Settingdistance
to 0 will set the motor to run indefinitely until stopped.Warning
Setting a
target_speed
higher than the maximum allowed will cause the method to throw an exception. To determine what the maximum possible target speed for the motor is, use themax_speed
method.Note
Note that for this method to move the wheel the expected
distance
, the correctwheel_diameter
value needs to be used.
- forward(target_speed, distance=0.0)[source]
Run the wheel forward at the desired speed in meters per second.
This method is a simple interface to move the motor that wraps a call to
set_target_speed
, specifying the forward direction.If desired, a
distance
to travel can also be specified in meters, after which the motor will stop. Settingdistance
to 0 will set the motor to run indefinitely until stopped.Note
Note that for this method to move the wheel the expected
distance
, the correctwheel_circumference
value needs to be used.
- backward(target_speed, distance=0.0)[source]
Run the wheel backwards at the desired speed in meters per second.
This method is a simple interface to move the wheel that wraps a call to
set_target_speed
, specifying the back direction.If desired, a
distance
to travel can also be specified in meters, after which the motor will stop. Settingdistance
to 0 will set the motor to run indefinitely until stopped.Note
Note that for this method to move the wheel the expected
distance
, the correctwheel_circumference
value needs to be used.
- property current_speed
Returns the speed currently being achieved by the motor in meters per second.
This value may differ from the target speed set through
set_target_speed
.
- property distance
Returns the distance the wheel has travelled in meters.
This value depends on the correct
wheel_circumference
value being set.
- property max_speed
The approximate maximum speed possible for the wheel attached to the motor shaft, given the motor specs, gear ratio and wheel circumference.
This value depends on the correct
wheel_circumference
value being set.
6.3.1. Parameters
6.4. LED
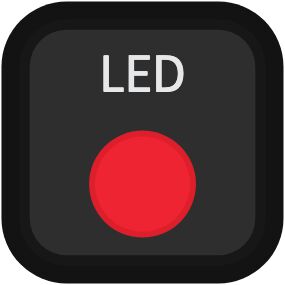
Note
This is a Digital Component which connects to a Digital Port [D0-D7].
from time import sleep
from pitop import LED
led = LED("D2")
led.on()
print(led.is_lit)
sleep(1)
led.off()
print(led.is_lit)
sleep(1)
led.toggle()
print(led.is_lit)
sleep(1)
print(led.value) # Returns 1 is the led is on or 0 if the led is off
- class pitop.pma.LED(*args, **kwargs)[source]
Encapsulates the behaviour of an LED.
An LED (Light Emitting Diode) is a simple light source that can be controlled directly.
- Parameters:
port_name (str) – The ID for the port to which this component is connected
name (str) – Component name, defaults to led. Used to access this component when added to a
pitop.Pitop
object.
- property own_state
Representation of an object state that will be used to determine the current state of an object.
- close()[source]
Shut down the device and release all associated resources. This method can be called on an already closed device without raising an exception.
This method is primarily intended for interactive use at the command line. It disables the device and releases its pin(s) for use by another device.
You can attempt to do this simply by deleting an object, but unless you’ve cleaned up all references to the object this may not work (even if you’ve cleaned up all references, there’s still no guarantee the garbage collector will actually delete the object at that point). By contrast, the close method provides a means of ensuring that the object is shut down.
For example, if you have a buzzer connected to port D0, but then wish to attach an LED instead:
>>> from pitop import Buzzer, LED >>> bz = Buzzer("D0") >>> bz.on() >>> bz.off() >>> bz.close() >>> led = LED("D0") >>> led.blink()
Device
descendents can also be used as context managers using thewith
statement. For example:>>> from pitop import Buzzer, LED >>> with Buzzer("D0") as bz: ... bz.on() ... >>> with LED("D0") as led: ... led.on() ...
- property active_high
When
True
, thevalue
property isTrue
when the device’spin
is high. WhenFalse
thevalue
property isTrue
when the device’s pin is low (i.e. the value is inverted).This property can be set after construction; be warned that changing it will invert
value
(i.e. changing this property doesn’t change the device’s pin state - it just changes how that state is interpreted).
- blink(on_time=1, off_time=1, n=None, background=True)
Make the device turn on and off repeatedly.
- Parameters:
on_time (float) – Number of seconds on. Defaults to 1 second.
off_time (float) – Number of seconds off. Defaults to 1 second.
n (int or None) – Number of times to blink;
None
(the default) means forever.background (bool) – If
True
(the default), start a background thread to continue blinking and return immediately. IfFalse
, only return when the blink is finished (warning: the default value of n will result in this method never returning).
- property closed
Returns
True
if the device is closed (see theclose()
method). Once a device is closed you can no longer use any other methods or properties to control or query the device.
- property config
Returns a dictionary with the set of parameters that can be used to recreate an object.
- static ensure_pin_factory()
Ensures that
Device.pin_factory
is set appropriately.This is called implicitly upon construction of any device, but there are some circumstances where you may need to call it manually. Specifically, when you wish to retrieve board information without constructing any devices, e.g.:
Device.ensure_pin_factory() info = Device.pin_factory.board_info
If
Device.pin_factory
is notNone
, this function does nothing. Otherwise it will attempt to locate and initialize a default pin factory. This may raise a number of different exceptions includingImportError
if no valid pin driver can be imported.
- classmethod from_config(config_dict)
Creates an instance of a Recreatable object with parameters in the provided dictionary.
- classmethod from_file(path)
Creates an instance of an object using the JSON file from the provided path.
- static import_class(module_name, class_name)
Imports a class given a module and a class name.
- property is_active
Returns
True
if the device is currently active andFalse
otherwise. This property is usually derived fromvalue
. Unlikevalue
, this is always a boolean.
- property is_lit
Returns
True
if the device is currently active andFalse
otherwise. This property is usually derived fromvalue
. Unlikevalue
, this is always a boolean.
- off()
Turns the device off.
- on()
Turns the device on.
- property pin
The
Pin
that the device is connected to. This will beNone
if the device has been closed (see theclose()
method). When dealing with GPIO pins, querypin.number
to discover the GPIO pin (in BCM numbering) that the device is connected to.
- print_config()
- print_state()
- save_config(path)
Stores the set of parameters to recreate an object in a JSON file.
- property source_delay
The delay (measured in seconds) in the loop used to read values from
source
. Defaults to 0.01 seconds which is generally sufficient to keep CPU usage to a minimum while providing adequate responsiveness.
- property state
Returns a dictionary with the state of the current object and all of its children.
- toggle()
Reverse the state of the device. If it’s on, turn it off; if it’s off, turn it on.
- property value
Returns 1 if the device is currently active and 0 otherwise. Setting this property changes the state of the device.
6.5. Light Sensor
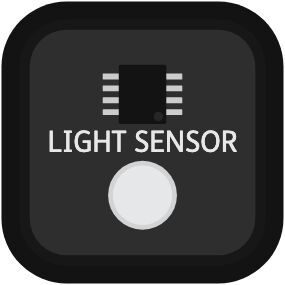
Note
This is a Analog Component which connects to a Analog Port [A0-A3].
from time import sleep
from pitop import LightSensor
light_sensor = LightSensor("A1")
while True:
# Returns a value depending on the amount of light
print(light_sensor.reading)
sleep(0.1)
- class pitop.pma.LightSensor(port_name, pin_number=1, name='light_sensor', number_of_samples=3)[source]
Encapsulates the behaviour of a light sensor module.
A simple analogue photo transistor is used to detect the intensity of the light striking the sensor. The component contains a photoresistor which detects light intensity. The resistance decreases as light intensity increases; thus the brighter the light, the higher the voltage.
Uses an Analog-to-Digital Converter (ADC) to turn the analog reading from the sensor into a digital value.
By default, the sensor uses 3 samples to report a
reading
, which takes around 0.5s. This can be changed by modifying the parameternumber_of_samples
in the constructor.- Parameters:
port_name (str) – The ID for the port to which this component is connected
number_of_samples (str) – Amount of sensor samples used to report a
reading
. Defaults to 3.name (str) – Component name, defaults to light_sensor. Used to access this component when added to a
pitop.Pitop
object.
- property reading
Take a reading from the sensor.
- Returns:
A value representing the amount of light striking the sensor at the current time from 0 to 999.
- Return type:
- property value
Get a simple binary value based on a reading from the device.
- Returns:
1 if the sensor is detecting any light, 0 otherwise
- Return type:
integer
- property own_state
Representation of an object state that will be used to determine the current state of an object.
6.6. Potentiometer
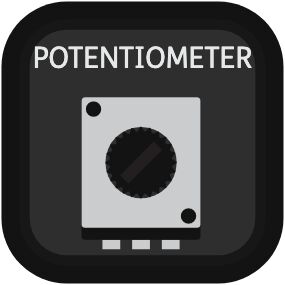
Note
This is a Analog Component which connects to a Analog Port [A0-A3].
from time import sleep
from pitop import Potentiometer
potentiometer = Potentiometer("A3")
while True:
# Returns the current position of the Potentiometer
print(potentiometer.position)
sleep(0.1)
- class pitop.pma.Potentiometer(port_name, pin_number=1, name='potentiometer', number_of_samples=1)[source]
Encapsulates the behaviour of a potentiometer.
A potentiometer is a three-terminal resistor with a sliding or rotating contact that forms an adjustable voltage divider. The component is used for measuring the electric potential (voltage) between the two ‘end’ terminals. If only two of the terminals are used, one end and the wiper, it acts as a variable resistor or rheostat. Potentiometers are commonly used to control electrical devices such as volume controls on audio equipment.
Uses an Analog-to-Digital Converter (ADC) to turn the analog reading from the sensor into a digital value.
- Parameters:
port_name (str) – The ID for the port to which this component is connected
number_of_samples (str) – Amount of sensor samples used to report a
position
. Defaults to 1.name (str) – Component name, defaults to potentiometer. Used to access this component when added to a
pitop.Pitop
object.
- property own_state
Representation of an object state that will be used to determine the current state of an object.
- property position
Get the current reading from the sensor.
- Returns:
A value representing the potential difference (voltage) from 0 to 999.
- Return type:
- property value
Get a simple binary value based on a reading from the device.
- Returns:
1 if the sensor is detecting a potential difference (voltage), 0 otherwise
- Return type:
integer
6.7. Servo Motor
Note
This is a Motor Component which connects to a ServoMotor Port [S0-S3].
from time import sleep
from pitop import ServoMotor, ServoMotorSetting
servo = ServoMotor("S0")
# Scan back and forward across a 180 degree angle range in 30 degree hops using default servo speed
for angle in range(90, -100, -30):
print("Setting angle to", angle)
servo.target_angle = angle
sleep(0.5)
# you can also set angle with a different speed than the default
servo_settings = ServoMotorSetting()
servo_settings.speed = 25
for angle in range(-90, 100, 30):
print("Setting angle to", angle)
servo_settings.angle = angle
servo.setting = servo_settings
sleep(0.5)
sleep(1)
# Scan back and forward displaying current angle and speed
STOP_ANGLE = 80
TARGET_SPEED = 40
print("Sweeping using speed ", -TARGET_SPEED)
servo.target_speed = -TARGET_SPEED
current_state = servo.setting
current_angle = current_state.angle
# sweep using the already set servo speed
servo.sweep()
while current_angle > -STOP_ANGLE:
current_state = servo.setting
current_angle = current_state.angle
current_speed = current_state.speed
print(f"current_angle: {current_angle} | current_speed: {current_speed}")
sleep(0.05)
print("Sweeping using speed ", TARGET_SPEED)
# you can also sweep specifying the speed when calling the sweep method
servo.sweep(speed=TARGET_SPEED)
while current_angle < STOP_ANGLE:
current_state = servo.setting
current_angle = current_state.angle
current_speed = current_state.speed
print(f"current_angle: {current_angle} | current_speed: {current_speed}")
sleep(0.05)
- class pitop.pma.ServoMotor(port_name, zero_point=0, name='servo')[source]
Represents a pi-top servo motor component.
Note that pi-top servo motors use an open-loop control system. As such, the output of the device (e.g. the angle and speed of the servo horn) cannot be measured directly. This means that you can set a target angle or speed for the servo, but you cannot read the current angle or speed.
- Parameters:
port_name (str) – The ID for the port to which this component is connected.
zero_point (int) – A user-defined offset from ‘true’ zero.
name (str) – Component name, defaults to servo. Used to access this component when added to a
pitop.Pitop
object.
- property own_state
Representation of an object state that will be used to determine the current state of an object.
- property zero_point
Represents the servo motor angle that the library treats as ‘zero’. This value can be anywhere in the range of -90 to +90.
For example, if the zero_point were set to be -30, then the valid range of values for setting the angle would be -60 to +120.
Warning
Setting a zero point out of the range of -90 to 90 will cause the method to raise an exception.
- property angle_range
Returns a tuple with minimum and maximum possible angles where the servo horn can be moved to.
If
zero_point
is set to 0 (default), the angle range will be (-90, 90).
- property setting
Returns the current state of the servo motor, giving current angle and current speed.
- Returns:
:class:’ServoMotorSetting` object that has angle and speed attributes.
- property current_angle
Returns the current angle that the servo motor is at.
Note
If you need synchronized angle and speed values, use
ServoMotor.state()
instead, this will return both current angle and current speed at the same time.- Returns:
float value of the current angle of the servo motor in degrees.
- property current_speed
Returns the current speed the servo motor is at.
Note
If you need synchronized angle and speed values, use
ServoMotor.state()
instead, this will return both current angle and current speed at the same time.- Returns:
float value of the current speed of the servo motor in deg/s.
- property smooth_acceleration
Gets whether or not the servo is configured to use a linear acceleration profile to ramp speed at start and end of cycle.
- Returns:
boolean value of the acceleration mode
- property target_angle
Returns the last target angle that has been set.
- Returns:
float value of the target angle of the servo motor in deg.
- property target_speed
Returns the last target speed that has been set.
- Returns:
float value of the target speed of the servo motor in deg/s.
- sweep(speed=None)[source]
Moves the servo horn from the current position to one of the servo motor limits (maximum/minimum possible angle), moving at the specified speed. The speed value must be a number from -100.0 to 100.0 deg/s.
The sweep direction is given by the speed.
Setting a
speed
value higher than zero will move the horn to the maximum angle (90 degrees by default), while a value less than zero will move it to the minimum angle (-90 degress by default).Warning
Using a
speed
out of the valid speed range will cause the method to raise an exception.
6.8. Sound Sensor
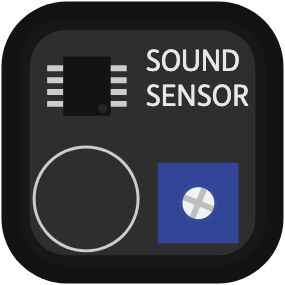
Note
This is a Analog Component which connects to a Analog Port [A0-A3].
from time import sleep
from pitop import SoundSensor
sound_sensor = SoundSensor("A2")
while True:
# Returns reading the amount of sound in the room
print(sound_sensor.reading)
sleep(0.1)
- class pitop.pma.SoundSensor(port_name, pin_number=1, name='sound_sensor', number_of_samples=1)[source]
Encapsulates the behaviour of a sound sensor.
A sound sensor component is typically a simple microphone that detects the vibrations of the air entering the sensor and produces an analog reading based on the amplitude of these vibrations.
Uses an Analog-to-Digital Converter (ADC) to turn the analog reading from the sensor into a digital value.
- Parameters:
port_name (str) – The ID for the port to which this component is connected
number_of_samples (str) – Amount of sensor samples used to report a
reading
. Defaults to 1.name (str) – Component name, defaults to sound_sensor. Used to access this component when added to a
pitop.Pitop
object.
- property reading
Take a reading from the sensor. Uses a builtin peak detection system to retrieve the sound level.
- Returns:
A value representing the volume of sound detected by the sensor at the current time from 0 to 500.
- Return type:
- property value
Get a simple binary value based on a reading from the device.
- Returns:
1 if the sensor is detecting any sound, 0 otherwise
- Return type:
integer
- property own_state
Representation of an object state that will be used to determine the current state of an object.
6.9. Ultrasonic Sensor
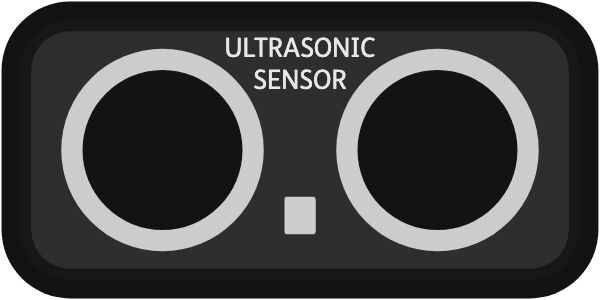
Note
This is a Digital Component which connects to a Digital Port [D0-D7].
from time import sleep
from pitop import UltrasonicSensor
distance_sensor = UltrasonicSensor("D3", threshold_distance=0.2)
# Set up functions to print when an object crosses 'threshold_distance'
distance_sensor.when_in_range = lambda: print("in range")
distance_sensor.when_out_of_range = lambda: print("out of range")
while True:
# Print the distance (in meters) to an object in front of the sensor
print(distance_sensor.distance)
sleep(0.1)
- class pitop.pma.UltrasonicSensor(port_name, queue_len=5, max_distance=3, threshold_distance=0.3, partial=False, name='ultrasonic')[source]
- property own_state
Representation of an object state that will be used to determine the current state of an object.
- property max_distance
The maximum distance that the sensor will measure in meters.
This value is specified in the constructor and is used to provide the scaling for the
value
attribute. Whendistance
is equal tomax_distance
,value
will be 1.
- property threshold_distance
The distance, measured in meters, that will trigger the
when_in_range
andwhen_out_of_range
events when crossed.This is simply a meter-scaled variant of the usual
threshold
attribute.
- property distance
Returns the current distance measured by the sensor in meters.
Note that this property will have a value between 0 and
max_distance
.
- property value
Returns a value between 0, indicating that something is either touching the sensor or is sufficiently near that the sensor can’t tell the difference, and 1, indicating that something is at or beyond the specified max_distance.
- property pin
- close()[source]
Shut down the device and release all associated resources. This method can be called on an already closed device without raising an exception.
This method is primarily intended for interactive use at the command line. It disables the device and releases its pin(s) for use by another device.
You can attempt to do this simply by deleting an object, but unless you’ve cleaned up all references to the object this may not work (even if you’ve cleaned up all references, there’s still no guarantee the garbage collector will actually delete the object at that point). By contrast, the close method provides a means of ensuring that the object is shut down.
For example, if you have a buzzer connected to port D0, but then wish to attach an LED instead:
>>> from pitop import Buzzer, LED >>> bz = Buzzer("D0") >>> bz.on() >>> bz.off() >>> bz.close() >>> led = LED("D0") >>> led.blink()
Device
descendents can also be used as context managers using thewith
statement. For example:>>> from pitop import Buzzer, LED >>> with Buzzer("D0") as bz: ... bz.on() ... >>> with LED("D0") as led: ... led.on() ...
- property in_range
- property when_in_range
- property when_out_of_range