9. API - pi-top Robotics
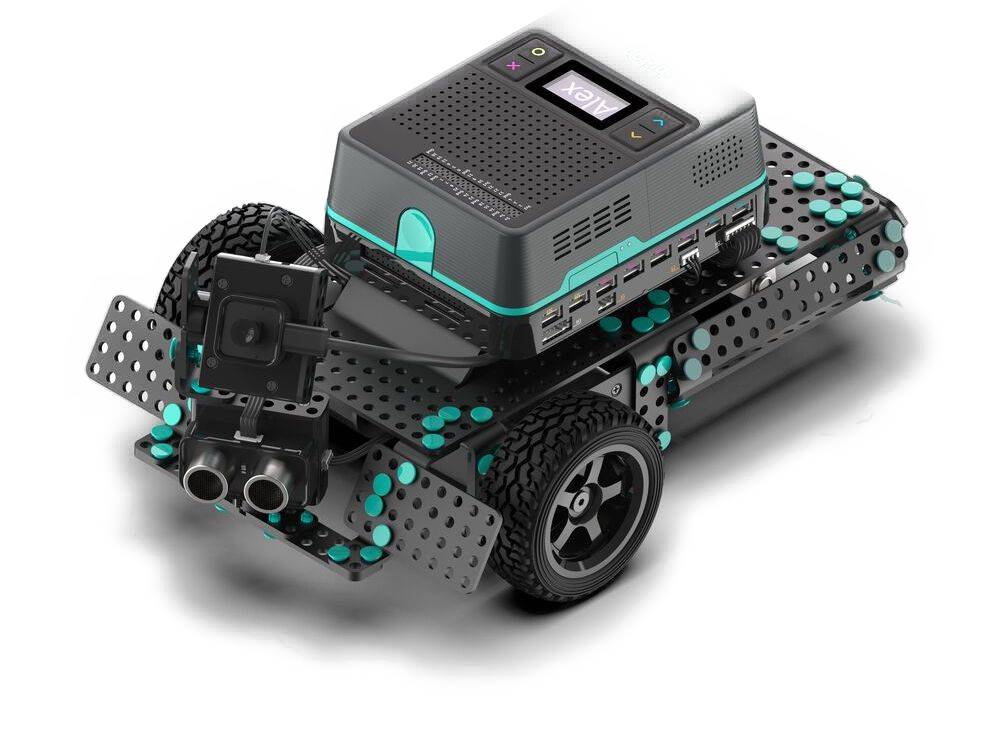
With the pi-top Robotics and Electronics Kits, you can build different types of robots. In this SDK, we provide a set of classes that represent some useful configurations.
9.1. Drive Controller
Note
This is a composite component that contains two EncoderMotor Components.
from time import sleep
from pitop.robotics import DriveController
drive = DriveController(left_motor_port="M3", right_motor_port="M0")
# Move forward
drive.forward(speed_factor=0.3)
# If a distance is not provided, the robot will move indefinitely
# Let it move for 1 second before stopping
sleep(1)
drive.stop()
# Rotate in place 180 degrees
drive.rotate(angle=180)
# Move to the right, following a curve for 10 centimeters
drive.right(speed_factor=0.5, turn_radius=0.5, distance=0.1)
# Move backward
drive.backward(speed_factor=0.5, distance=0.1)
- class pitop.robotics.DriveController(left_motor_port: str = 'M3', right_motor_port: str = 'M0', name: str = 'drive')[source]
Represents a vehicle with two wheels connected by an axis, and an optional support wheel or caster.
- robot_move(linear_speed: int | float, angular_speed: int | float, turn_radius: int | float = 0.0, distance: int | float | None = None) None [source]
- forward(speed_factor: int | float, hold: bool = False, distance: int | float | None = None) None [source]
Move the robot forward.
- Parameters:
speed_factor (float) – Factor relative to the maximum motor speed, used to set the velocity, in the range -1.0 to 1.0. Using negative values will cause the robot to move backwards.
hold (bool) – Setting this parameter to true will cause subsequent movements to use the speed set as the base speed.
distance (float) – Distance to travel in meters. If not provided, the robot will move indefinitely
- backward(speed_factor: int | float, hold: bool = False, distance: int | float | None = None) None [source]
Move the robot backward.
- Parameters:
speed_factor (float) – Factor relative to the maximum motor speed, used to set the velocity, in the range -1.0 to 1.0. Using negative values will cause the robot to move forwards.
hold (bool) – Setting this parameter to true will cause subsequent movements to use the speed set as the base speed.
distance (float) – Distance to travel in meters. If not provided, the robot will move indefinitely
- left(speed_factor: int | float, turn_radius: int | float = 0, distance: int | float | None = None) None [source]
Make the robot move to the left, using a circular trajectory.
- Parameters:
speed_factor (float) – Factor relative to the maximum motor speed, used to set the velocity, in the range -1.0 to 1.0. Using negative values will cause the robot to turn right.
turn_radius (float) – Radius used by the robot to perform the movement. Using turn_radius=0 will cause the robot to rotate in place.
distance (float) – Distance to travel in meters. If not provided, the robot will move indefinitely
- right(speed_factor: int | float, turn_radius: int | float = 0, distance: int | float | None = None) None [source]
Make the robot move to the right, using a circular trajectory.
- Parameters:
speed_factor (float) – Factor relative to the maximum motor speed, used to set the velocity, in the range -1.0 to 1.0. Using negative values will cause the robot to turn left.
turn_radius (float) – Radius used by the robot to perform the movement. Using turn_radius=0 will cause the robot to rotate in place.
distance (float) – Distance to travel in meters. If not provided, the robot will move indefinitely
- target_lock_drive_angle(angle: int | float) None [source]
Make the robot move in the direction of the specified angle, while maintaining the current linear speed.
- rotate(angle: int | float, time_to_take: int | float | None = None, max_speed_factor: float = 0.3) None [source]
Rotate the robot in place by a given angle and stop.
- Parameters:
angle (float) – Angle of the turn.
time_to_take (float) – Expected duration of the rotation, in seconds. If not provided, the motors will perform the rotation using % of the maximum angular speed allowed by the motors, to ensure the robot can perform the rotation without issues.
max_speed_factor (bool) – Factor relative to the maximum motor speed, used to set the velocity, in the range 0 to 1.0.
- stop_rotation() None [source]
Stop any angular movement performed by the robot.
In the case where linear and rotational movements are being performed at the same time (e.g.: during a left turn with a turn radius different to 0), calling this method will cause the robot to continue the linear movement, so it will continue to move forward.
9.2. Pan Tilt Controller
Note
This is a composite component that contains two ServoMotor Components.
- class pitop.robotics.PanTiltController(servo_pan_port='S0', servo_tilt_port='S3', name='pan_tilt')[source]
Represents a pan-tilt using two servo motors.
- CALIBRATION_FILE_NAME = 'pan_tilt.conf'
- property pan_servo
- property tilt_servo
- property track_object: PanTiltObjectTracker
- calibrate(save=True, reset=False)[source]
Calibrates the assembly to work in optimal conditions.
Based on the provided arguments, it will either load the calibration values stored in the pi-top, or it will run the calibration process, requesting the user input in an interactive fashion.
- Parameters:
reset (bool) – If true, the existing calibration values will be reset, and the calibration process will be started. If set to false, the calibration values will be retrieved from the calibration file.
save (bool) – If reset is true, this parameter will cause the calibration values to be stored to the calibration file if set to true. If save=False, the calibration values will only be used for the current session.
9.3. Pincer Controller
Note
This is a composite component that contains two ServoMotor Components.
- class pitop.robotics.PincerController(left_pincer_port='S3', right_pincer_port='S0', name='pincers')[source]
Represents a pincer that uses two servo motors connected parallel to each other.
- CALIBRATION_FILE_NAME = 'pincers.conf'
- calibrate(save=True, reset=False)[source]
Calibrates the assembly to work in optimal conditions. Based on the provided arguments, it will either load the calibration values stored in the pi-top, or it will run the calibration process, requesting the user input in an interactive fashion.
- Parameters:
reset (bool) – If true, the existing calibration values will be reset, and the calibration process will be started. If set to false, the calibration values will be retrieved from the calibration file.
save (bool) – If reset is true, this parameter will cause the calibration values to be stored to the calibration file if set to true. If save=False, the calibration values will only be used for the current session.